· articles · 4 min read
By Jason LCORS / Preflight - Explained With Example (2024)
Delve deep into Cross-Origin Resource Sharing (CORS), definition, the security issues it tackles, how it works at the HTTP protocol level, common developer mistakes, etc.
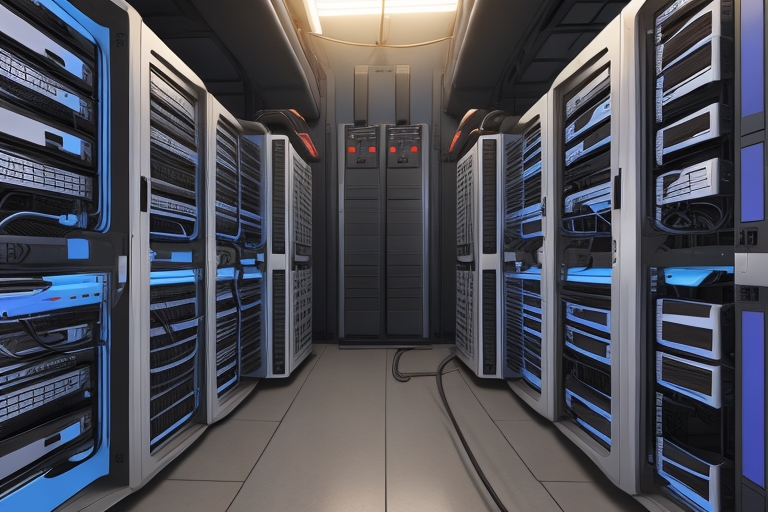
What is CORS?
CORS stands for Cross-Origin Resource Sharing. It’s a security feature implemented by web browsers to protect users from malicious websites trying to perform unauthorized actions on behalf of the user. CORS defines how web servers can specify who can access their resources and under what conditions.
Consider an example: Imagine you have a web application running at https://app.example.com, and you want to fetch data from an API hosted at https://api.exampleapi.com. Without CORS, browsers would block this request by default, due to the “same-origin policy” that restricts web pages from making requests to domains other than their own. CORS is the mechanism that allows https://app.example.com to request data from https://api.exampleapi.com.
Security Issues Addressed by CORS
CORS primarily addresses the security issue of Cross-Site Request Forgery (CSRF) and Cross-Site Scripting (XSS).
CSRF: Without CORS, malicious websites could make requests to other sites on behalf of the user, potentially performing harmful actions like changing account settings or making purchases.
XSS: Malicious scripts injected into a web page could make unauthorized requests to other sites, potentially compromising user data or security.
How CORS Works
CORS operates at the HTTP protocol level, utilizing special headers to facilitate communication between web servers and browsers. Here’s how it works:
Browser Sends a Request: When a web page at one origin (e.g., https://app.example.com) makes a request to another origin (e.g., https://api.exampleapi.com), the browser attaches the Origin header with the requesting page’s origin.
Server Responds with CORS Headers: The server receives the request and determines whether to allow it based on its CORS configuration. If allowed, it responds with CORS headers, such as Access-Control-Allow-Origin, specifying which origins are permitted to access its resources.
Example Request:
GET /data HTTP/1.1
Host: api.exampleapi.com
Origin: https://app.example.com
Example Response:
HTTP/1.1 200 OK
Access-Control-Allow-Origin: https://app.example.com
How do I enable CORS on a server?
Enabling CORS (Cross-Origin Resource Sharing) on your server involves configuring your server to include the necessary HTTP headers that allow cross-origin requests from specified origins.
NodeJs
- Install
cors
middleware using npm.
npm install cors
- In your Express.js application, import and use the cors middleware:
const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for a specific origin (e.g., https://example.com)
app.use(cors({
origin: 'https://example.com',
methods: ['GET', 'POST'], // Specify the HTTP methods allowed
}));
// ...your routes and other middleware...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code configures CORS to allow cross-origin requests from https://example.com and specifies which HTTP methods are allowed.
ASP.Net
In an ASP.NET Core application, you can enable CORS in the Startup.cs file:
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.DependencyInjection;
public void ConfigureServices(IServiceCollection services)
{
// Add CORS policy with specific origins and methods
services.AddCors(options =>
{
options.AddPolicy("AllowSpecificOrigin", builder =>
{
builder.WithOrigins("https://example.com")
.AllowAnyMethod()
.AllowAnyHeader();
});
});
// ...
}
public void Configure(IApplicationBuilder app)
{
// Use the CORS policy
app.UseCors("AllowSpecificOrigin");
}
In this example, CORS is configured to allow cross-origin requests from https://example.com with any HTTP method and headers.
Python
- In a Django project, you can enable CORS using the django-cors-headers package. First, install it using pip:
pip install django-cors-headers
- Add corsheaders to your Django project’s INSTALLED_APPS in the settings.py file:
INSTALLED_APPS = [
# ...
'corsheaders',
# ...
]
- Configure CORS settings in settings.py:
CORS_ALLOWED_ORIGINS = [
"https://example.com", # Specify the allowed origin(s)
]
# Optional: Allow specific HTTP methods and headers
CORS_ALLOW_METHODS = ['GET', 'POST']
CORS_ALLOW_HEADERS = ['Content-Type']
This code configures CORS to allow cross-origin requests from https://example.com and specifies the allowed HTTP methods and headers.
Common Developer Mistakes
Developers often encounter CORS-related issues during development. Let’s address some common questions and mistakes:
FAQ
Why do I still see CORS errors even after configuring my server correctly?
CORS policies must be set on both the server and the client. Ensure that your client-side code (e.g., JavaScript) includes the correct origin when making requests and that the server is configured to accept requests from that origin.
What is a preflight request, and why is it important?
Preflight requests are OPTIONS requests that the browser sends to check if a cross-origin request is allowed. They help ensure the safety of certain HTTP methods and headers. Your server must handle these requests correctly.
Can I override CORS restrictions for testing purposes?
Yes, but be cautious. Browsers offer extensions and command-line flags (e.g., —disable-web-security in Chrome) for testing, but these should not be used in production or on untrusted websites.
Further Reading
In my opinion, StackOverflow is a great resource for know the intrinsic details about any topic. Here are some of the most commonly asked questions.