· articles · 3 min read
By Ankit JainHow do I enable CORS on JSON-SERVER?
CORS (Cross-Origin Resource Sharing) is enabled by default on JSON-SERVER. However, if you need to customize CORS options, you can use middleware to modify the CORS headers.
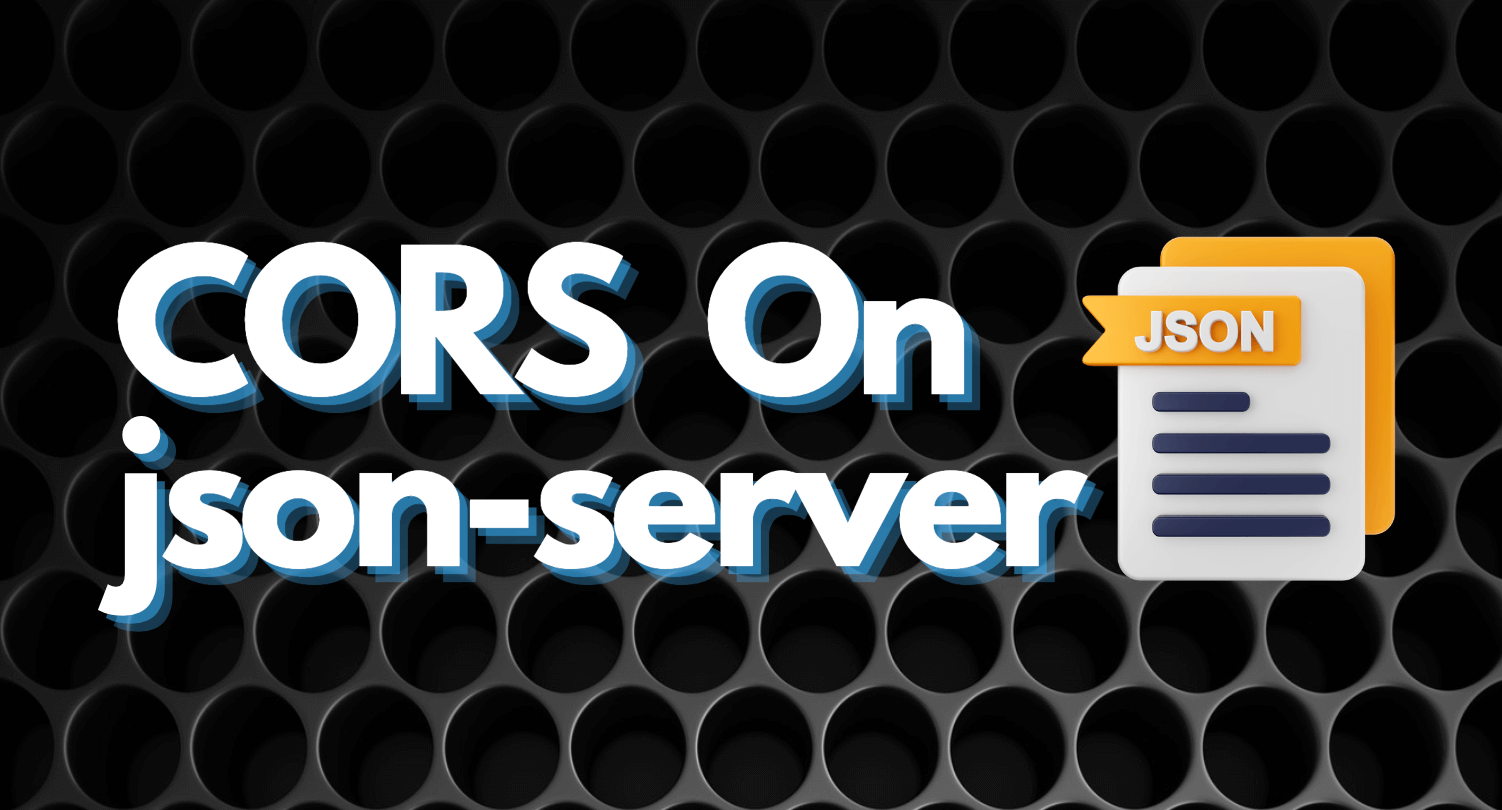
Cross-Origin Resource Sharing (CORS) is a security feature available in all modern web browsers to make the web secure. This works by restricting unauthenticated usage of cross domain HTTP requests. However, during development, especially when working with APIs like JSON-SERVER, it’s common to encounter CORS errors if your front-end application is served from a different port or domain than your back-end server.
JSON-SERVER, a common tool for prototyping APIs, supports configuring CORS out of the box with the help of @tinyhttp/cors package. This write-up will guide you through customizing CORS on JSON-SERVER, allowing you to make cross-origin requests seamlessly during development.
Default Support
JSON SERVER inherently enables CORS, requiring no additional setup from your end. It automatically handles preflight requests (OPTIONS
) by responding with a 200 OK status and includes a Access-Control-Allow-Origin: *
header in the response.
The wildcard *
simplifies matters in most scenarios, yet it falls short when dealing with CORS requests that include credentials. In my experience, attempting such a request resulted in an error:
Access to XMLHttpRequest at ‘http://localhost:3000/posts’ from origin ‘https://www.test-cors.org’ has been blocked by CORS policy: The value of the ‘Access-Control-Allow-Origin’ header in the response must not be the wildcard ’*’ when the request’s credentials mode is ‘include’. The credentials mode of requests initiated by the XMLHttpRequest is controlled by the withCredentials attribute.
A straightforward workaround involves encapsulating JSON SERVER within middleware, thereby manually setting the necessary HTTP headers to accommodate CORS effectively. This approach is elaborated in the next section.
Customizing CORS
The default CORS configuration of JSON-SERVER works for most of the development scenarios. However, you might need to customize CORS behavior. For instance, you may want to limit the origins that can request your server or customize headers and methods allowed. This customization can be achieved by using middleware in a custom server setup.
Step 1: Install JSON-SERVER Globally
If you haven’t already, install JSON-SERVER globally using npm:
npm install -g json-server
Step 2: Create a Custom Server File
Instead of starting JSON-SERVER directly from the command line with the default settings, create a JavaScript file to configure your server, for example, server.js:
const jsonServer = require('json-server');
const server = jsonServer.create();
const router = jsonServer.router('db.json'); // Your database file
const middlewares = jsonServer.defaults();
// Add custom middleware for CORS
server.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', '*'); // Allow any origin
res.header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, PATCH, OPTIONS');
res.header('Access-Control-Allow-Headers', 'Origin, X-Requested-With, Content-Type, Accept');
next();
});
server.use(router);
server.listen(3000, () => {
console.log('JSON Server is running');
});
In this file, jsonServer.router('db.json')
points to your database file, and jsonServer.defaults()
returns the default middlewares used by JSON-SERVER, which includes static files server, logger, and others including the CORS middleware.
The line which is setting header Access-Control-Allow-Origin
should be adjusted according to your Origin
or the source of the API requests. You can further customize it to allow multiple domain by setting, or picking the Origin
from the original request.
res.header('Access-Control-Allow-Origin', 'https://some-domain.com'); // Allow specific domain
res.header('Access-Control-Allow-Origin', req.headers['origin'] || req.get('Origin');); // Allow any domain coming in Origin header.
Step 3: Run Your Custom Server
To start your JSON-SERVER with custom CORS settings, run your server.js file with Node.js:
node server.js
This setup starts your JSON-SERVER with CORS enabled, allowing requests from any origin. You can adjust the Access-Control-Allow-Origin
header to restrict it to specific domains, enhancing security for production environments.