· articles · 6 min read
By Sudar Selva Ganesh MREST API Versioning: A Comprehensive Guide for Developers
Explore REST API versioning—why it's essential for managing breaking changes, ensuring backward compatibility, and evolving APIs smoothly. Learn strategies, best practices, and techniques like URI paths, headers, and semantic versioning.
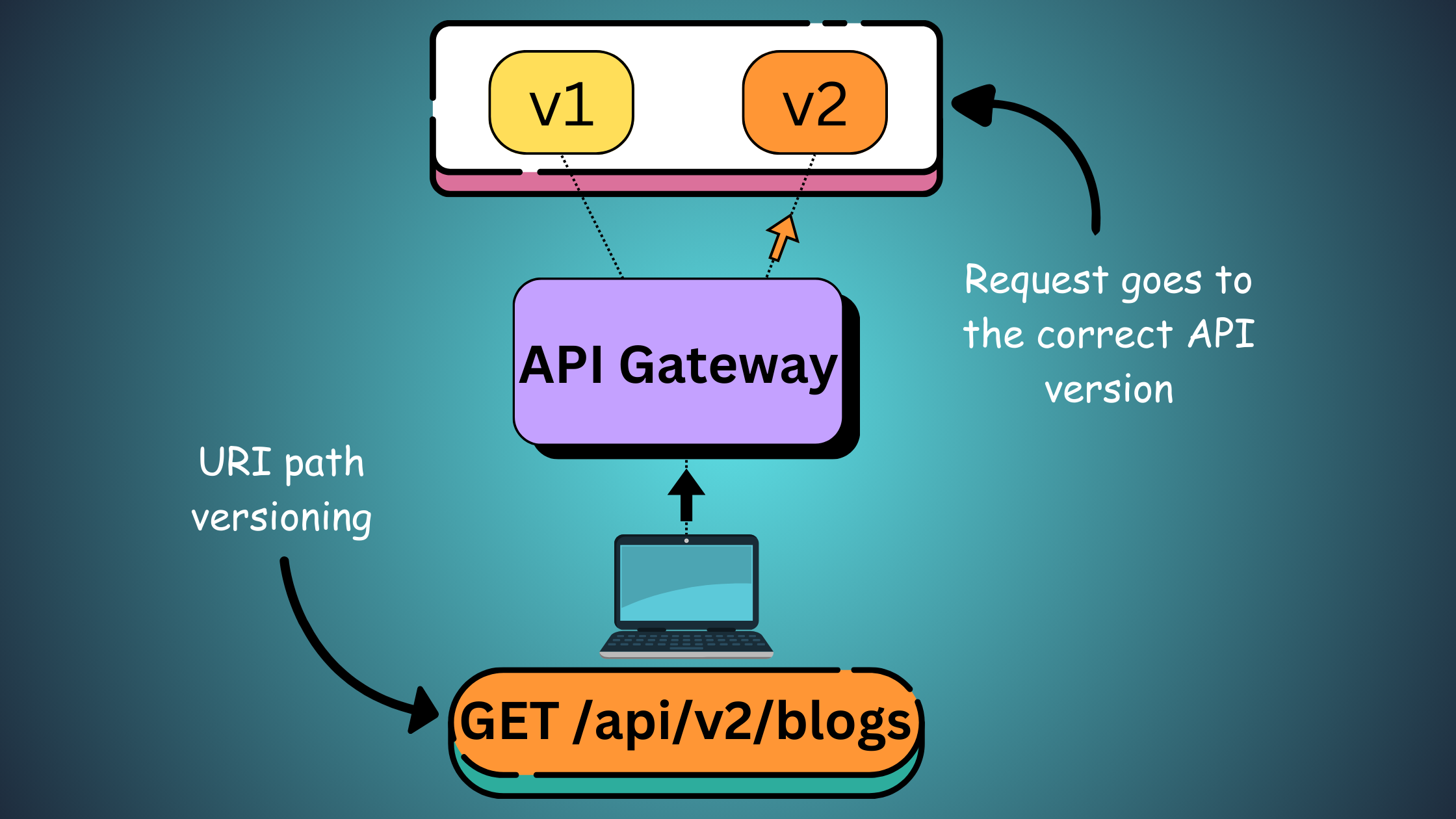
If you’re a developer working on REST APIs, you know that change is inevitable. Whether it’s adding new features, fixing bugs, or enhancing the architecture, updates to your API are part of the job. But these changes can have a big impact on users who rely on your API.
That’s where API versioning comes in—it’s a way to manage changes without breaking things for existing users. In this guide, you will know what API versioning is, why it’s so important, and how you can implement it effectively in your projects. Let’s dive in!
Why REST API Versioning Matters?
When you change an API, even small updates can disrupt applications that depend on it. Imagine removing a field or altering a response format—it could break functionality for your users, especially if they weren’t expecting it. API versioning solves this by letting you introduce changes while preserving older versions for users who need them.
Versioning allows you to:
- Avoid Breaking Changes: Keep existing functionality intact for current users.
- Support Incremental Evolution: Add new features or fixes without disrupting older workflows.
- Provide Clear Communication: Indicate which features are stable, deprecated, or experimental.
Without versioning, maintaining backward compatibility becomes a guessing game, and you risk frustrating your users.
Key Concepts in REST API Versioning
Before diving into implementation, it’s essential to understand the core principles of API versioning:
Backward Compatibility: Changes should not affect existing integrations. For example, adding a new field is fine, but removing one isn’t.
Breaking Changes: When changes are unavoidable (e.g., renaming a field or altering response structures), a new version is necessary to avoid disruptions.
Deprecation Policies: Communicate changes early, provide clear timelines, and help users migrate to newer versions.
Version Identification: Make the version explicit, so users know which version they’re interacting with.
Strategies for REST API Versioning
There’s no one-size-fits-all approach to API versioning. The right strategy depends on your API’s requirements and how you plan to handle changes. Below are the most commonly used techniques:
1. URI Path Versioning
In this method, the API version number is embedded in the URL of the endpoint. For example, clients interested in retrieving all products from a database would make a request to an endpoint. This method is widely regarded as one of the most popular and straightforward techniques for API versioning.
https://example.com/v1/products
Pros:
- Simple and explicit.
- Easy to identify the version from the request.
Cons:
- Versions are tightly coupled to the URL structure.
- Updates require users to change endpoint URLs.
2. Query Parameter Versioning
Query Parameter Versioning is a simple way for APIs to handle different versions. You can add a version number as a query parameter in the URL, so you can tell the API which version you want to use.
https://api.example.com/resource?version=1
Pros:
- Keeps the base URL consistent.
- Useful for non-breaking updates.
Cons:
- Less visible compared to URI path versioning.
- Parsing query parameters can add complexity.
3. Header Versioning
Header Versioning is an approach for versioning APIs where the version number is specified in the HTTP headers of the request rather than the URL path. This method is often used when you want to keep the URL clean and prevent clutter while still allowing multiple versions of the API to coexist.
Pros:
- Keeps the URL clean.
- Flexible for content negotiation.
Cons:
- Harder for users to discover.
- More challenging to debug during development.
Not sure when to introduce a new version?
Not every change requires a new version. Understanding when to version is key to maintaining a stable and usable API. Here are some scenarios:
When you don’t need new version:
- Adding New Endpoints: Introducing new routes doesn’t affect existing functionality.
- Adding Optional Fields: Including additional data in responses won’t break old integrations if they ignore unknown fields.
- Non-breaking Bug Fixes: Correcting logic or improving performance can usually be done without versioning.
When you need new version:
- Removing Fields or Endpoints: If users rely on the removed elements, this change is breaking.
- Renaming Fields: Changing a field name will break integrations expecting the old name.
- Altering Response Structures: Significant changes to response formats require a new version.
Best Practices for REST API Versioning
Implementing a versioning strategy is only half the battle. Following these best practices will help you manage versions effectively:
1. Plan for Versioning Early
Even if you don’t version your initial release, design your API with future versions in mind. Use consistent naming conventions and avoid tightly coupling functionality to specific implementation details.
2. Document Changes Thoroughly
Your API documentation should clearly describe:
Version-specific changes: List functionality, endpoints, or data structures that changed between versions and explain what’s new or different for the user.
Deprecation notices: Note that advance warnings of feature or version retirement must be given to users so they can make appropriate plans.
Migration guides: Give guidelines to help the user transition easily from one version to another.
3. Use Semantic Versioning Principles
Adopt a structured versioning scheme, such as:
- Major: Introduce breaking changes (e.g., v1 → v2).
- Minor: Add new features that don’t break existing functionality.
- Patch: Apply non-breaking bug fixes.
4. Provide Deprecation Warnings
Notify users when you plan to deprecate a version. Include:
- Deprecation timelines.
- Warnings in API responses.
- Guidance for migrating to newer versions.
5. Automate Testing Across Versions
Set up automated tests for all active versions to ensure updates don’t introduce regressions. Test both the new version and older ones simultaneously.
Managing Legacy Versions
Over time, older API versions become harder to maintain. Here’s how you can manage legacy versions effectively:
- Track Usage: Use analytics to monitor which versions are actively in use.
- Communicate Clearly: Announce when a version is deprecated and provide timelines for sunsetting it.
- Provide Grace Periods: Allow users enough time to migrate before removing support.
- Introduce Soft Deprecation: Add warnings or limit new features to current versions before fully deprecating older ones.
Example: Evolving a REST API
Let’s say you have a simple API endpoint:
{
"id": 1,
"field1": "value1",
"field2": "value2"
}
Scenario 1: Non-breaking Update
You add a new field:
{
"id": 1,
"field1": "value1",
"field2": "value2",
"field3": "value3"
}
Currently, the existing users continue to work without any issues, as the new field is optional.
Scenario 2: Breaking Change
You rename field2 to contact_field. This change breaks clients expecting field2.
{
"id": 1,
"field1": "value1",
"contact_field": "value2"
}
To handle this, release a new version:
GET /v2/resources
Update your documentation to explain the changes and provide a migration guide for users.
Conclusion
As a developer, your understanding of REST API versioning is a critical part of building scalable and reliable API services. A clear versioning strategy communicates the maturity of your product and minimizes disruptions for your users. Whether you choose URI paths, headers, or another method, the key is to plan carefully, communicate effectively, and provide the tools users need to adapt. Remember to be backward compatible always.
Your knowledge will evolve continuously, and so your API design skills. With proper versioning, you can ensure that evolution is smooth and predictable—for both you and the developers relying on your work.