· articles · 3 min read
By Jason LSecure Your RESTful API: A Practical Guide
Discover effective techniques and real-world examples for securing REST APIs. Learn how to protect your REST APIs from vulnerabilities and ensure robust API security with practical insights and best practices.
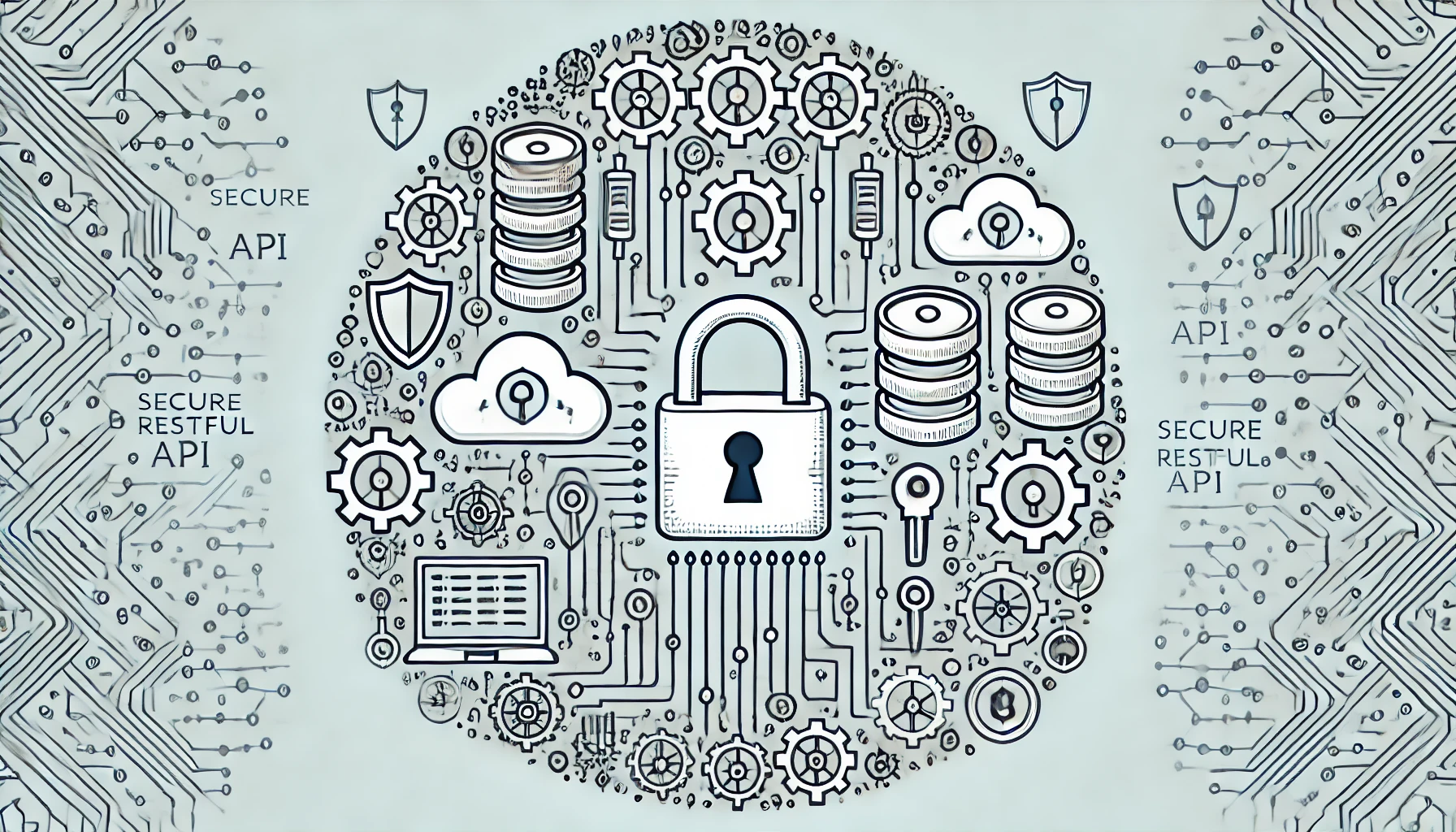
Protecting your RESTful API shields sensitive data and keeps unauthorized users out. Let’s dive into practical ways to secure your API with real implementation examples.
1. Protect APIs With Authentication
API Keys
API keys work as simple access credentials. Each client gets their own unique key to include in requests. Your server checks this key before granting access.
Example:
- Key: c6a7b6e1-3d75-4fc0-bf8c-712a46a9e8d2
- Include it in your request header:
GET /api/resource
Host: yourapi.com
X-API-Key: c6a7b6e1-3d75-4fc0-bf8c-712a46a9e8d2
See it in action: Stripe APIs
OAuth 2.0
OAuth 2.0 lets apps access resources securely without exposing passwords. It controls what third parties can access.
Example: Use Google login:
- Users log in through Google
- Get an OAuth token
- Send this token with API requests
JWT (JSON Web Tokens)
JWTs pack user info into three parts: header, payload, and signature. The signature verifies everything stays intact.
Example: Send JWT tokens in headers after login:
GET /api/resource
Host: yourapi.com
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
Check out: Auth0 Management API
Basic Authentication
Send username and password with each request. Simple but effective when implemented right.
Example:
GET /api/resource
Host: yourapi.com
Authorization: Basic Base64EncodedCredentials
See: GitHub REST API
OAuth 2.0 with Bearer Tokens
Works like JWT - get a token through OAuth and include it in request headers.
Example: GitHub OAuth 2.0
SSL Certificate
Both sides verify each other’s certificates before talking. This creates mutual trust and blocks man-in-the-middle attacks.
Benefits:
- Proves client identity conclusively
- Stops sneaky attacks in transit
- Builds two-way trust between systems
2. Role-Based Access Control (RBAC)
RBAC limits what users can do based on their assigned roles. Each role gets specific permissions.
Example: Employee Management System roles:
- Admin: Create, read, update, delete all records
- Manager: View and update records only
- Employee: View only their own record
- HR: Update personal info but not salary details
Implementation:
- Alice (HR manager) gets “HR” and “Manager” roles
- Bob (employee) only gets the “Employee” role
- Your API checks roles before allowing actions
3. IP Whitelisting
Only allow requests from trusted IP addresses. Block everything else.
How it works:
- List approved IP addresses
- Configure your API to check incoming IPs
- Reject any requests from unlisted IPs
Example: B2B setup with two customers:
- Customer A: 203.0.113.12
- Customer B: 198.51.100.15
Only these IPs can access your API - everyone else gets blocked.
4. Rate Limiting
Control how many requests clients can make using standard headers:
- X-RateLimit-Limit
- X-RateLimit-Remaining
- X-RateLimit-Reset
Example: Cap clients at 100 requests per minute.
5. HTTPS Encryption
Encrypt all traffic between clients and your server. Force secure connections with:
Strict-Transport-Security: max-age=31536000; includeSubDomains; preload
Example: Install SSL/TLS on your server. Stripe requires this for all API calls.
6. CORS (Cross-Origin Resource Sharing)
Specify which domains can access your API from browsers.
Example: Configure headers to allow only trusted websites to call your API.
Conclusion
Secure your API based on your specific needs. The examples above show real-world implementations you can adapt. Pick the right mix of methods, implement them carefully, and sleep better knowing your API stands strong against threats.